[
debug
linux
optimize
reverse-design
]
23 Oct 2020
function calling status tracing
use linux utilities valgrind (it also can analyze memory leakage) and kcachegrind ui tool to help us analyze function usage status
1. install utilities
in ubuntu system, use the command to install these utilities:
sudo apt install kcachegrind valgrind
2. run the target process
use the valgrind to generate report for calling usage status
valgrind --tool=callgrind <process>
after the command, the report file likes “callgrind.out." should be generated in your folder
the report document has lots of information, we can use the utility kcachegrind to help us show these information:
kcachegrind callgrind.out.<pid>
[
QT
javascript
c++
]
03 Apr 2019
QML and C++
while creating a Qt quick application, it is impossible to avoid communicate between qml and c++, it can be shown like:
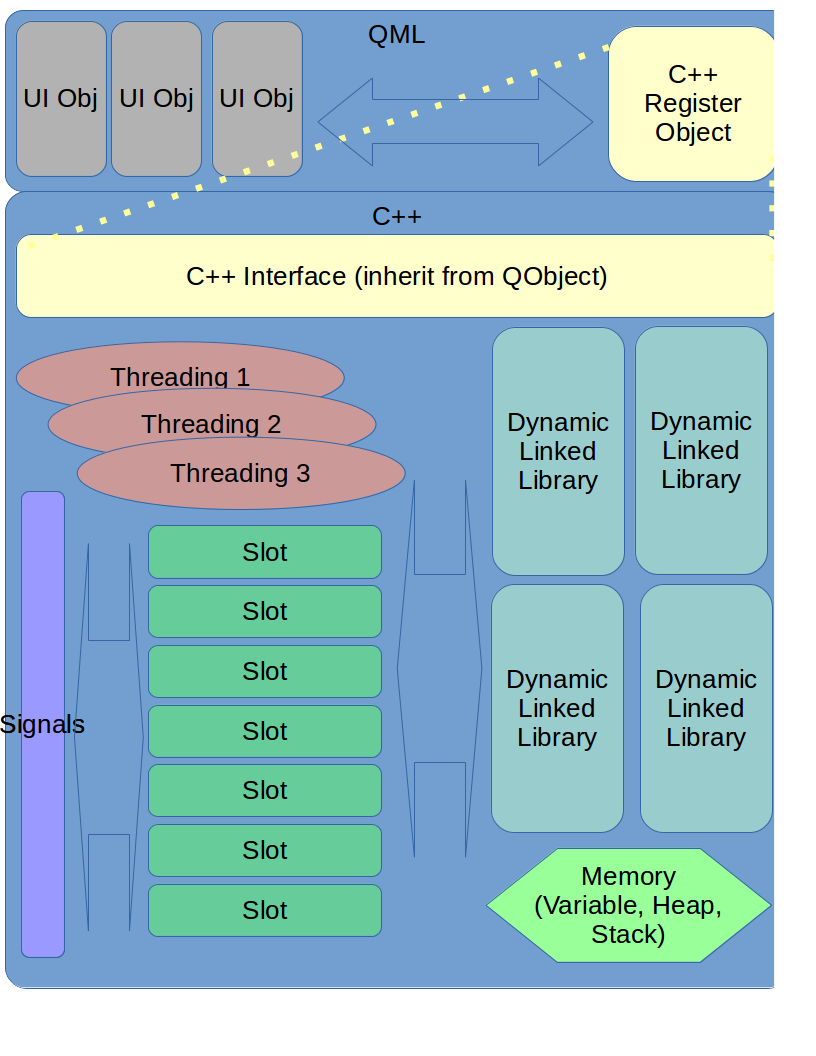
there are some methods, and I like the first one:
call c++ code from qml
create object
- a class is inherited from QObject
you need create a c++ object and inherited from QObject:
class CInterface : public QObject {
...
}
- register class in main.cpp
after you created the class, you need register it in main.cpp
#include <QQmlEngine>
...
qmlRegisterType<CInterface>("interface", 1, 0, "interface");
- use in qml
finally, you just add the code in your qml file like:
import interface 1.0
...
interface {
...
}
communicate between them
- QT signal and slot
- c++ signal to qml
we assume our object has a signal sigAction like this:
class CInterface : public QObject {
...
signals:
void sigAction();
}
then we use it in qml:
interface {
id: c_interface
onSigAction: {
...
}
}
- qml signal to c++
we assume our object has a slot slotAction in C++ and a signal sigQml in QML:
class CInterface : public QObject {
...
public slots:
void slotAction();
}
and the QML part:
interface {
id: c_interface
}
...
Component.onCompleted: {
c_interface.slotAction();
}
- Q_INVOKABLE declaration
the Q_INVOKABLE declaration can let QML call c++ method directly:
(c++ part)
class CInterface : public QObject {
...
public:
Q_INVOKABLE void action();
}
and the QML part:
interface {
id: c_interface
}
...
Component.onCompleted: {
c_interface.action();
}
type between qml and c++
there are usually used types between c++ and qml, you can refer here to find you want to use:
Qt Type |
QML Type |
bool |
bool |
unsigned int, int |
int |
double |
double |
float, qreal |
real |
QString |
string |
QVariant |
var |
call qml from c++
in this case, you have to set the objectName for the object you want to call from c++
Rectangle {
id: "rect"
objectName: "rectObj"
...
}
then you can find the object from c++
QQuickItem *item = engine.findChild<QQuickItem*>("rectObj");